Learn Python Programming Language with Examples
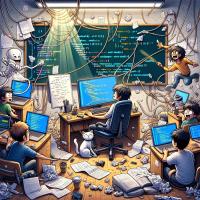
Python is one of the most popular and versatile programming languages today. Its simplicity and readability make it an excellent choice for beginners, while its powerful libraries and frameworks make it indispensable for experienced developers. This article introduces you to Python programming with practical examples, covering basic concepts that will get you started on your programming journey.
Introduction to Python
Python was created by Guido van Rossum and released in 1991. It is a high-level, interpreted language known for its clear syntax and code readability. Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. It is widely used in web development, data analysis, artificial intelligence, scientific computing, and more.
Getting Started with Python
Before diving into the examples, make sure you have Python installed on your computer. You can download the latest version from the official Python website (python.org). Python comes with an interactive interpreter, allowing you to execute Python code directly in your terminal or command prompt.
Hello, World! Example
Let's start with the classic "Hello, World!" program. This example demonstrates how to print a string of text in Python.
pythonprint("Hello, World!")
When you run this script, Python will output:
Hello, World!
Variables and Data Types
Variables are used to store information that can be referenced and manipulated in a program. Python is dynamically-typed, meaning you don't need to declare the type of variable ahead of time.
pythonname = "John Doe"
age = 30
height = 5.9
is_adult = True
print(name, "is", age, "years old.")
This code snippet defines variables of different data types and prints them.
Lists and Loops
Lists are ordered collections that can hold a variety of data types. Loops, such as the for
loop, allow you to iterate over a sequence.
pythonfruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print("I like", fruit)
This loop will print each item in the fruits
list.
Functions
Functions are blocks of reusable code that perform a specific task. Define a function using the def
keyword.
pythondef greet(name):
print("Hello,", name + "!")
greet("Alice")
This function, greet
, takes a name as an argument and prints a greeting.
Conditional Statements
Conditional statements, using if
, elif
, and else
, allow you to execute different blocks of code based on certain conditions.
pythonage = 18
if age < 18:
print("You are a minor.")
elif age == 18:
print("Congratulations on becoming an adult!")
else:
print("You are an adult.")
This code will print a message based on the value of age
.
Working with Libraries
Python's strength lies in its extensive standard library and third-party libraries. For example, you can use the requests
library to make HTTP requests.
pythonimport requests
response = requests.get('https://api.github.com')
print(response.status_code)
First, you'll need to install the requests
library using pip: pip install requests
. This code sends a GET request to GitHub's API and prints the HTTP status code of the response.
Conclusion
This article provided a brief introduction to Python with simple examples covering basic concepts. Python's simplicity, readability, and vast ecosystem make it an excellent language for beginners and professionals alike. As you continue to explore Python, you'll discover a world of possibilities, from web development and data analysis to machine learning and beyond. Happy coding!